Defines methods called when the target is stopped. More...
#include <IGDBTarget.h>
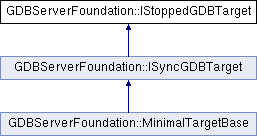
Public Member Functions | |
virtual const PlatformRegisterList * | GetRegisterList ()=0 |
Returns a pointer to a persistent list of the registers supported by the target. | |
virtual GDBStatus | ReadFrameRelatedRegisters (int threadID, RegisterSetContainer ®isters)=0 |
Reads program counter, stack pointer and stack base pointer registers. | |
virtual GDBStatus | ReadTargetRegisters (int threadID, RegisterSetContainer ®isters)=0 |
Reads the values of all registers from the target. | |
virtual GDBStatus | WriteTargetRegisters (int threadID, const RegisterSetContainer ®isters)=0 |
Writes one or more target registers. | |
virtual GDBStatus | ReadTargetMemory (ULONGLONG Address, void *pBuffer, size_t *pSizeInBytes)=0 |
Reads the memory of the underlying target. | |
virtual GDBStatus | WriteTargetMemory (ULONGLONG Address, const void *pBuffer, size_t sizeInBytes)=0 |
Writes the memory of the underlying target. | |
virtual GDBStatus | GetDynamicLibraryList (std::vector< DynamicLibraryRecord > &libraries)=0 |
Fills the list of the dynamic libraries currently loaded in the target. | |
virtual GDBStatus | GetThreadList (std::vector< ThreadRecord > &threads)=0 |
Fills the list of the threads currently present in the target. | |
virtual GDBStatus | SetThreadModeForNextCont (int threadID, DebugThreadMode mode, OUT bool *pNeedRestoreCall, IN OUT INT_PTR *pRestoreCookie)=0 |
Sets the mode in which an individual thread will continue before the next debug event. | |
virtual GDBStatus | Terminate ()=0 |
Terminates the target. | |
virtual GDBStatus | CreateBreakpoint (BreakpointType type, ULONGLONG Address, unsigned kind, OUT INT_PTR *pCookie)=0 |
Sets a breakpoint at a given address. | |
virtual GDBStatus | RemoveBreakpoint (BreakpointType type, ULONGLONG Address, INT_PTR Cookie)=0 |
Removes a previously set breakpoint. | |
virtual GDBStatus | ExecuteRemoteCommand (const std::string &command, std::string &output)=0 |
This handler is invoked when user sends an arbitrary command to the GDB stub ("mon <command>" in GDB). | |
virtual IFLASHProgrammer * | GetFLASHProgrammer ()=0 |
Returns a pointer to an IFLASHProgrammer instance, or NULL if not supported. The returned instance should be persistent (e.g. the same object that implements IStoppedGDBTarget). | |
virtual | ~IStoppedGDBTarget () |
Detailed Description
Defines methods called when the target is stopped.
This interface defines the methods of the GDB target that are called when the target is stopped. Actual targets should implement the ISyncGDBTarget interface instead.
Constructor & Destructor Documentation
|
inlinevirtual |
Member Function Documentation
|
pure virtual |
Sets a breakpoint at a given address.
- Parameters
-
type Specifies the type of the supported breakpoint. Address Specifies the address where the breakpoint should be set. kind Specifies the additional information provided by GDB. For data breakpoints this is the length of the watched region. pCookie receives an arbitrary INT_PTR value that will be passed to RemoveBreakpoint() once this breakpoint is removed.
- Remarks
- It is not necessary to implement this method for software breakpoints. If GDB encounters a "not supported" reply, it will set the breakpoint using the WriteTargetMemory() call. It is only recommended to implement this method for the software breakpoints if it can set them better than GDB itself.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
This handler is invoked when user sends an arbitrary command to the GDB stub ("mon <command>" in GDB).
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Fills the list of the dynamic libraries currently loaded in the target.
If the target supports dynamic libraries (a.k.a DLLs, a.k.a. shared libraries, a.k.a. shared objects), this method should provide the information about them by filling the libraries vector.
- Parameters
-
libraries Contains an empty vector that should be filled with instances of DynamicLibraryRecord
- Returns
- If the target does not support dynamic libraries, the method should return kGDBNotSupported. Refer to GDBStatus documentation for more information.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Returns a pointer to an IFLASHProgrammer instance, or NULL if not supported. The returned instance should be persistent (e.g. the same object that implements IStoppedGDBTarget).
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Returns a pointer to a persistent list of the registers supported by the target.
This method should return a list to a constant global PlatformRegisterList instance containing the descriptions of all the registers present in the target.
The method is called during initialization and the table returned by it should be present during the entire target lifetime.
|
pure virtual |
Fills the list of the threads currently present in the target.
If the target supports multiple threads, this method should provide the information about them by filling the threads vector.
- Parameters
-
threads Contains an empty vector that should be filled with instances of ThreadRecord
- Returns
- If the target does not support multiple threads, the method should return kGDBNotSupported. Refer to GDBStatus documentation for more information.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Reads program counter, stack pointer and stack base pointer registers.
- Returns
- See the GDBStatus description for a list of valid return codes.
- Parameters
-
threadID Specifies the ID of the thread to query registers Contains the storage for the register values. The storage is initialized with RegisterValue instances flagged as invalid and sizes set based on the GetRegisterList() call.
- Remarks
- If this method returns an error, GDB will read all registers using the ReadTargetRegisters() method. Thus it only makes sense to implement this method if reading frame-related registers instead of all registers saves time.
Here's an example implementation of this method:
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Reads the memory of the underlying target.
|
pure virtual |
Reads the values of all registers from the target.
This method should read all registers from the target (or a single target thread). See the ReadFrameRelatedRegisters() method description for implementation examples.
|
pure virtual |
Removes a previously set breakpoint.
This method should remove a breakpoint previously set with CreateBreakpoint().
- Parameters
-
type Equal to the type argument of the corresponding CreateBreakpoint() call. Address Equal to the Address argument of the corresponding CreateBreakpoint() call. Cookie contains the arbitrary INT_PTR value provided by the CreateBreakpoint() method.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Sets the mode in which an individual thread will continue before the next debug event.
This method allows setting different continuation modes (e.g. single-step, free run or halt) for different threads of a multi-threaded program. If the target does not support it, the method should return kGDBNotSupported.
- Parameters
-
threadID Specifies the ID of the thread that is being controlled mode Specifies the mode in which the thread should be put until the next debug event pNeedRestoreCall Set the value pointed by this argument to TRUE if this method needs to be called again with mode == dtmRestore once the next debug event completes. pRestoreCookie If mode is not dtmRestore, you set pNeedRestoreCall to TRUE, this argument can receive an arbitrary INT_PTR value that will be passed to the method later during the dtmRestore call. If mode is dtmRestore, this argument points to the previously saved value.
- Remarks
- Before the method is actually used, it is called with mode == dtmProbe and an invalid threadID to determine whether the target supports it. Thus, if mode is dtmProbe, the threadID argument should be ignored and the method should immediately return either kGDBNotSupported or kGDBSuccess.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Terminates the target.
Implemented in GDBServerFoundation::MinimalTargetBase.
|
pure virtual |
Writes the memory of the underlying target.
|
pure virtual |
Writes one or more target registers.
This method should write the target registers that have valid values provided.
- Parameters
-
threadID Specifies the ID of the thread to query registers Provides the values for the registers to write. The size of the registers collection can be determined by calling the RegisterSetContainer::RegisterCount() method. If an i-th register needs to be modified, the registers[i].Valid field is set.
- Remarks
- Here's an example implementation: {for (size_t i = 0; i < registers.RegisterCount(); i++){if (!registers[i].Valid)continue;m_pTargetImpl->WriteRegister(i, registers[i].ToUInt32());}return kGDBSuccess;}
The documentation for this class was generated from the following file: