Implements supported gdbserver packets by invoking methods of a provided IGDBTarget object. More...
#include <GDBStub.h>
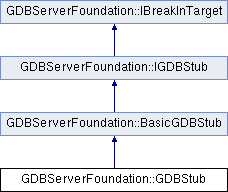
Public Member Functions | |
GDBStub (ISyncGDBTarget *pTarget, bool own=true) | |
~GDBStub () | |
virtual void | OnBreakInRequest () |
virtual StubResponse | Handle_QueryStopReason () |
virtual StubResponse | Handle_g (int threadID) |
Returns the values of all target registers in one block. | |
virtual StubResponse | Handle_G (int threadID, const BazisLib::TempStringA ®isterValueBlock) |
Sets the value of all target registers. | |
virtual StubResponse | Handle_P (int threadID, const BazisLib::TempStringA ®isterIndex, const BazisLib::TempStringA ®isterValue) |
Sets the value of exactly one register. | |
virtual StubResponse | Handle_m (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &length) |
Reads target memory. | |
virtual StubResponse | Handle_M (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &length, const BazisLib::TempStringA &data) |
Writes target memory. | |
virtual StubResponse | Handle_X (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &length, const BazisLib::TempStringA &binaryData) |
Writes target memory, data is transmitted in binary format. | |
virtual StubResponse | Handle_qXfer (const BazisLib::TempStringA &object, const BazisLib::TempStringA &verb, const BazisLib::TempStringA &annex, const BazisLib::TempStringA &offset, const BazisLib::TempStringA &length) |
virtual StubResponse | HandleRequest (const BazisLib::TempStringA &requestType, char splitterChar, const BazisLib::TempStringA &requestData) |
Handles a fully unescaped RLE-expanded request from GDB. | |
virtual StubResponse | Handle_c (int threadID) |
Continue executing selected thread. | |
virtual StubResponse | Handle_s (int threadID) |
Single-step selected thread. | |
virtual StubResponse | Handle_qfThreadInfo () |
Return a list of all thread IDs. | |
virtual StubResponse | Handle_qsThreadInfo () |
virtual StubResponse | Handle_qThreadExtraInfo (const BazisLib::TempStringA &strThreadID) |
Return user-friendly thread description. | |
virtual StubResponse | Handle_T (const BazisLib::TempStringA &strThreadID) |
Check whether the specified thread is alive. | |
virtual StubResponse | Handle_qC () |
Returns the current thread ID. | |
virtual StubResponse | Handle_vCont (const BazisLib::TempStringA &arguments) |
Sets step mode for each thread independently and continues execution. | |
virtual StubResponse | Handle_k () |
Kills the process. | |
virtual StubResponse | Handle_Zz (bool setBreakpoint, char type, const BazisLib::TempStringA &addr, const BazisLib::TempStringA &kind, const BazisLib::TempStringA &conditions) |
Sets or removes a breakpoint. | |
virtual StubResponse | Handle_qCRC (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &length) |
Computes CRC of a given memory block. | |
virtual StubResponse | Handle_qRcmd (const BazisLib::TempStringA &command) |
Executes an arbitrary target command sent by GDB. | |
virtual StubResponse | Handle_vFlashErase (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &length) |
virtual StubResponse | Handle_vFlashWrite (const BazisLib::TempStringA &addr, const BazisLib::TempStringA &binaryData) |
virtual StubResponse | Handle_vFlashDone () |
![]() | |
BasicGDBStub () | |
![]() | |
virtual | ~IGDBStub () |
Protected Member Functions | |
virtual BazisLib::DynamicStringA | BuildGDBReportByName (const BazisLib::TempStringA &name, const BazisLib::TempStringA &annex) |
RegisterSetContainer | InitializeRegisterSetContainer () |
void | ResetAllCachesWhenResumingTarget () |
void | ProvideThreadInfo () |
![]() | |
virtual StubResponse | Handle_qSupported (const BazisLib::TempStringA &requestData) |
Stores features supported by GDB and reports features supported by the stub. | |
virtual StubResponse | Handle_H (const BazisLib::TempStringA &requestType) |
Sets thread ID for subsequent thread-related commands. | |
StubResponse | StopRecordToStopReply (const TargetStopRecord &rec, const char *pReportedRegisterValues=NULL, bool updateLastReportedThreadID=true) |
int | GetThreadIDForOp (bool isRegOp) |
void | AppendRegisterValueToString (const RegisterValue &val, size_t sizeInBytes, BazisLib::DynamicStringA &str, const char *pSuffix=NULL) |
StubResponse | FormatGDBStatus (GDBStatus status) |
void | RegisterStubFeature (const char *pFeature) |
Additional Inherited Members | |
![]() | |
int | m_ThreadIDForCont |
int | m_ThreadIDForReg |
int | m_LastReportedCurrentThreadID |
Detailed Description
Implements supported gdbserver packets by invoking methods of a provided IGDBTarget object.
- Examples:
- SimpleWin32Server/SimpleWin32Server.cpp.
Constructor & Destructor Documentation
GDBServerFoundation::GDBStub::GDBStub | ( | ISyncGDBTarget * | pTarget, |
bool | own = true |
||
) |
|
inline |
Member Function Documentation
|
protectedvirtual |
|
virtual |
Continue executing selected thread.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Returns the values of all target registers in one block.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Sets the value of all target registers.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Kills the process.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Reads target memory.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Writes target memory.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Sets the value of exactly one register.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Returns the current thread ID.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Computes CRC of a given memory block.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Return a list of all thread IDs.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Executes an arbitrary target command sent by GDB.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Return user-friendly thread description.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
|
virtual |
Single-step selected thread.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Check whether the specified thread is alive.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Sets step mode for each thread independently and continues execution.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Writes target memory, data is transmitted in binary format.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Sets or removes a breakpoint.
Implements GDBServerFoundation::BasicGDBStub.
|
virtual |
Handles a fully unescaped RLE-expanded request from GDB.
Reimplemented from GDBServerFoundation::BasicGDBStub.
|
protected |
|
inlinevirtual |
Implements GDBServerFoundation::IBreakInTarget.
|
protected |
|
protectedvirtual |
Reimplemented from GDBServerFoundation::BasicGDBStub.
The documentation for this class was generated from the following files: